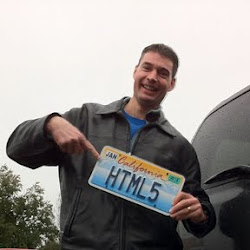
Peter Lubbers
Program Manager, Chrome Developer Relations
Google
Photo by +Guy Kawasaki
Peter Lubbers
Program Manager, Chrome Developer Relations
Google
Photo by +Guy Kawasaki
Evolution Not Revolution.
Who is communicating?
An origin consists of three parts:
Scheme, Host, and Port
# The following origins are all different # (default HTTP ports 80 and 443 are used if nothing is specified): http://www.example.com http://img.example.com https://www.example.com http://www.example.com:9999
# The origin is taken literally by browsers. For example: http://localhost:9999 #is not the same as: htpp://127.0.0.1:9999
# Note the new WebSocket schemes (80 and 443 are the default ports): ws://websockets.org wss://websockets.org
Without any security model, user agents might undertake actions detrimental to the user or to other parties. Over time, many web-related technologies have converged towards a common security model known colloquially as the same-origin policy.
With XHR and CORS, you receive data instead of [JSONP] code, which you can parse safely
Browser to Server:
HTTP POST /main HTTP/1.1 ... Referer: http://www.example.com/slides Origin: http://www.example.com
Server to Browser:
# Space separated origin list # Important: Think twice before adding * Access-Control-Allow-Origin: http://www.example.com Access-Control-Allow-Credentials: true
Web Intents
{ "name": "An app", "version": "1", "intents": { "http://webintents.org/save": [{ "type":["image/*"], "href":"/save.html", "disposition": "inline" }] } }
<intent action="http://webintents.org/save" type="image/*" href="save.html" disposition="inline" />
if(window.webkitIntent) { var i = window.webkitIntent; if(i.action == "http://webintents.org/save") { if(i.type == "image/png") { img.src = i.data; // the data } } }
How is communication established?
Cross Document Messaging
postMessage
APImessage
event listeners (use an origin white list)postMessage()
Sending messages:
worker.postMessage('Hello World'); window.postMessage(JSON.stringify({msg: 'Hello World'}), 'http://www.example.com');
Receiving messages:
window.addEventListener("message", processMessage,true); function processMessage(e) { if (e.origin == "http://www.example.com") { // Only process from trusted source! processMessage(e.data); } } // Some more exciting options later...
XMLHttpRequest Level 2
loadstart, progress, error, abort, load, loadend
responseType
text, blob, document, arraybuffer*
XMLHttpRequest Level 2
function upload(blobOrFile) { var xhr = new XMLHttpRequest(); //Can send cross domain now xhr.open('POST', '/server', true); xhr.onload = function(e) { ... }; xhr.send(blobOrFile); }
Server-Sent Events
eventSource
APIServer-Sent Events
//Create new EventSource var dataStream = new EventSource("http://www.example.com"); // Add handlers for open and error: dataStream.onopen = function(evt) { console.log("open"); } dataStream.onerror = function(evt) { console.log("error"); }; // Handle messages dataStream.onmessage = function(evt) { // Process message console.log("message: " + evt.data); }
WebSocket
So, what about SPDY?
"I use the metaphor of hammers and screwdrivers. Both tools are indispensible in my workshop...
Use the right tool for the job.
In the case of page and object delivery use SPDY.
In the case of lightweight or streaming data delivery look to WebSocket."
WebSocket
// Create new WebSocket, connect to ws://echo.websocket.org var exampleSocket = new WebSocket("ws://echo.websocket.org"); // Send messages: exampleSocket.send("Hello world"); // Listen for messages exampleSocket.onmessage = function (event) { console.log(event.data); } // Add listeners for the other events: exampleSocket.onopen = function(evt) { onOpen(evt) }; exampleSocket.onclose = function(evt) { onClose(evt) }; exampleSocket.onerror = function(evt) { onError(evt) };
WebRTC
MediaStream
and PeerConnection
Javascript APIsWhat is communicated?
postMessage()
1. Basic strings
worker.postMessage('Hello World'); window.postMessage(JSON.stringify({msg: 'Hello World'}), 'http://www.example.com');
2. JSON
worker.postMessage({msg: 'Look! no strings attached.'}, 'http://www.example.com');
postMessage()
3. Complex types (File
, Blob
, ArrayBuffer
) via structured cloning
var data = [1, 2, 3, 4]; // Blob() in Chrome 20, FF 13 worker.postMessage(new Blob(data.toString(), {type: 'text/plain'})); var uint8Array = new Uint8Array(data); worker.postMessage(uint8Array); worker.postMessage(uint8Array.buffer);
Transferable Objects
Same old friend, different semantics:
// Note: Prefixed for the moment worker.webkitPostMessage(arrayBuffer, [arrayBuffer]); window.webkitPostMessage(arrayBuffer, targetOrigin, [arrayBuffer]);
ArrayBuffer
) is transfered to new context (e.g. worker/window). Source buffer becomes unavailable.var uint8Array = new Uint8Array(1024 * 1024 * 32); // 32MB // Fill'r up! for (var i = 0, len = uint8Array.length; i < len; ++i) { uint8Array[i] = i; } worker.(uint8Array, [uint8Array.buffer]);
Pass multiple buffers at once and/or additional data:
worker.({ view1: uInt8Array, buffer2: anotherBuffer, username: 'johndoe' }, [uInt8Array.buffer, anotherBuffer]);
Questions?
Free monthly events, livestreamed and video taped
Follow the Chrome Developers Channel Live!